Well if you are iOS Developer with the swift starter as knowledge or you are coder that want to know the basic of objective-c view, this post is suitable for you. I will explain about anatomy of objective-c, encapsulation, and instance variable vs obj-c properties, setter getter on objective-c. I hope this post will help you to understand the basic of objective c. this post also suitable with you who want to start learning objective c.
Basically when you create a objective-c class (cocoa class) you will got two file, they are .h file extensions class and .m file extensions class. two classes is represents of objective-c class. each of them have different and specifics purpose.
The .h file is a header file, this file purpose is for the definition and identity of the class and to send information to the world. if you want to put property or method which open and accessed in the world (other classes) you should put that property here , that's the jobs of the header file.
the .m file is implementation file, this file purpose is doing implementation in the class, so you can put and do your private instance, private method logic and implementation from the header here.
Encapsulation is one of the oop (object oriented programing) concept, the simple definition is when you need to hiding or showing some of your instance, field, method to other classes or package. you can check here if you need detail explanation about it check here Encapsulation.
As I mention before in the anatomy of objective-c that the header file is for showing to the world and .m is for implementation. basically thing that I could say is :
In objective c there are two type of field, they are objective- c properties and instance variable. each them have different usability.
here the example of instance variable vs objective c properties
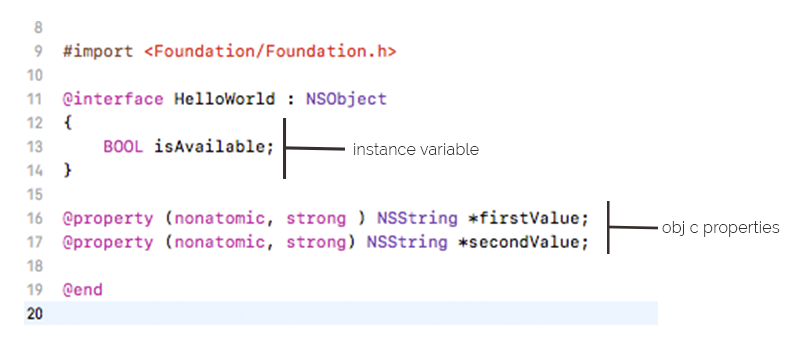
By default when you create objective-c properties on your header file, that's automatically create three element. they are instance variable, setter, getter.
The next question is, where I should use instance variable or using objective-c properties as variable. not all developers do this (will do all with objective-c properties) , but good developers know how to do this well. so if you are gonna access that field only on the implementation class use instance variable, and if you want to access it in other classes use objective-c properties.
That's all my short tutorials and basic things that you should know about objective C. I'll explain more on the next tutorial if possible, if you feel this tutorials help you feel free to share. thanks!
- Anatomy of Objective-C
Basically when you create a objective-c class (cocoa class) you will got two file, they are .h file extensions class and .m file extensions class. two classes is represents of objective-c class. each of them have different and specifics purpose.
The .h file is a header file, this file purpose is for the definition and identity of the class and to send information to the world. if you want to put property or method which open and accessed in the world (other classes) you should put that property here , that's the jobs of the header file.
the .m file is implementation file, this file purpose is doing implementation in the class, so you can put and do your private instance, private method logic and implementation from the header here.
Picture 1. Class structure (obj-c vs swift)
- Encapsulation
Encapsulation is one of the oop (object oriented programing) concept, the simple definition is when you need to hiding or showing some of your instance, field, method to other classes or package. you can check here if you need detail explanation about it check here Encapsulation.
As I mention before in the anatomy of objective-c that the header file is for showing to the world and .m is for implementation. basically thing that I could say is :
- .h file = public property, public method, instance variable
- .m file = private instance, private method, and implementation from header file.
- Objective c properties vs Instance variable.
In objective c there are two type of field, they are objective- c properties and instance variable. each them have different usability.
here the example of instance variable vs objective c properties
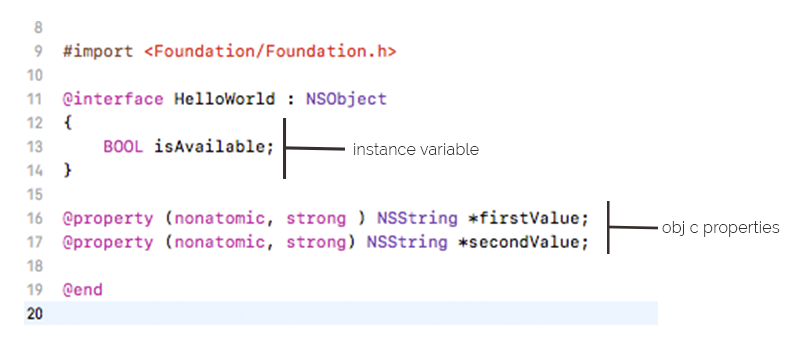
Picture 2 . Instance Variable & Objective C
By default when you create objective-c properties on your header file, that's automatically create three element. they are instance variable, setter, getter.
- Instance variable
Picture 3. Accessing instance variable on implementation class
- Setter and getter
Picture 4. Accessing setter and getter method of obj-c properties
The next question is, where I should use instance variable or using objective-c properties as variable. not all developers do this (will do all with objective-c properties) , but good developers know how to do this well. so if you are gonna access that field only on the implementation class use instance variable, and if you want to access it in other classes use objective-c properties.
That's all my short tutorials and basic things that you should know about objective C. I'll explain more on the next tutorial if possible, if you feel this tutorials help you feel free to share. thanks!